gs gs111 Types of Classes (Using Java) - Object Class - Quiz No.1
gs gs111 OOP Object Oriented Programming Java Quiz
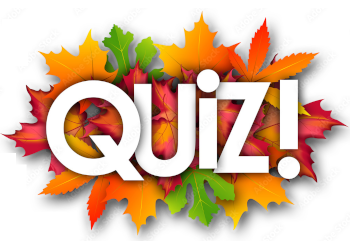
This quiz belongs to book/course code gs gs111 OOP Object Oriented Programming Java of gs organization. We have 178 quizzes available related to the book/course OOP Object Oriented Programming Java. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Types of Classes (Using Java). NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
class StudentHashcode { static int num = 60; int temp; Student() { temp = num; } public int hashCode() { return temp; } public static void main(String args[]) { Student s = new Student(); System.out.println(s.hashCode()); System.out.println(s); } }
60 60
3c Student@3c
3c Student@60
60 Student@3c
class Clock { public static void main(String[] args) { Clock time = new Clock(); System.out.println("" + time.getClass()); Integer i = new Integer(5); System.out.println("" + i.getClass()); } }
class Clock class java.lang.Integer
class Clock class java.lang.Datatype
class time class java.io.Integer
class Clock class java.io.Integer
class FormDetails { int id; String name; String tag; Details(int id, String name, String tag) { this.id=id; this.name=name; this.tag=tag; } public static void main(String args[]) { Details obj1=new Details(1,"Pawan","NoTag"); Details obj2=new Details(2,"Mahesh","NoTag"); System.out.println(obj1); System.out.println(obj2); } }
1 Pawan NoTag 2 Mahesh NoTag
1,"Pawan","NoTag" 2,"Mahesh","NoTag"
Details@15db9742 Details@6d06d69c
obj1@15db9742 obj2@6d06d69c
class PersonalDetails { String name; int age; Person (String name, int age) { this.name = name; this.age = age; } public String toString() { return name + " " + age; } public static void main(String[] args) { Person b = new Person("Gautam Nanda", 21); System.out.println(b.toString()); } }
class FinaliseObject { public void finalize() { System.out.println("This is the finalize() method "); } public static void main(String[] args) { String str = new String("Hi, Welcome in Java World"); str = null; System.gc(); System.out.println("This is finalize class"); } }
class Cloning implements Cloneable { int id; String name; Cloning(int id, String name) { this.id=id; this.name=name; } public Object clone()throws CloneNotSupportedException { return super.clone(); } public static void main(String args[]) { try { Cloning obj1=new Cloning(1,"dravid"); Cloning obj2=(Cloning)obj1.clone(); System.out.println(obj1.id+" "+obj1.name); System.out.println(obj2.id+" "+obj2.name); } catch(CloneNotSupportedException c){} } }
1 dravid 1 dravid
1 dravid 1 dravid 1 dravid
public class JavaClassExample { public static void main(String[] args) { Object obj1 = new String("Apple"); Class b = obj1.getClass(); System.out.println("Class is:" + b.getName()); } }
class IntegerHashCode { public static void main(String[] args) { int hashValue = Integer.hashCode("155"); System.out.println("Hash code Value for object is: " + hashValue); } }