gs gs111 Inheritance (Using Java) - Types of Inheritance - Quiz No.1
gs gs111 OOP Object Oriented Programming Java Quiz
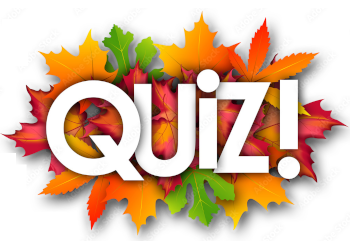
This quiz belongs to book/course code gs gs111 OOP Object Oriented Programming Java of gs organization. We have 178 quizzes available related to the book/course OOP Object Oriented Programming Java. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Inheritance (Using Java). NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
public class Inheritance { public static void main(String[] args) { Super i = new Super(); i.show(); } } class Super { public void show() { System.out.println("Base"); } } class Sub extends Super { public void show() { System.out.println("Derived"); } }
class Base { public void show() { System.out.println("Base"); } } class Derived extends Base { public void show() { System.out.println("Derived"); } } public class Inheritance1 { public static void main(String[] args) { Base i = new Base(); i.show(); } }
public class Inherit1 { public static void main(String[] args) { Base b = new Derived(); b.print(); } } class Base { public static void print() { System.out.println("Base"); } } class Derived extends Base { public static void print() { System.out.println("Derived class"); } }
class Base { public static void display() { System.out.println("Base"); } } class Derived extends Base { public static void display() { System.out.println("Derived"); } } class Inheritance2 { public static void main(String[] args) { Base x = new Base(); Base y = new Derived(); Derived z = new Derived(); x.display(); y.display(); z.display(); } }
Base Derived Derived
Derived Derived Base
Base Base Derived
class A { int a = 10; } class B extends A { int b = 20; } class C extends A { int b = 30; } class D extends B,C { void disp() { System.out.println(b); } } public class Display { public static void main(String[] args) { D d = new D(); d.calculate(); } }