gs gs111 Types of Classes (Using Java) - Base Class - Quiz No.1
gs gs111 OOP Object Oriented Programming Java Quiz
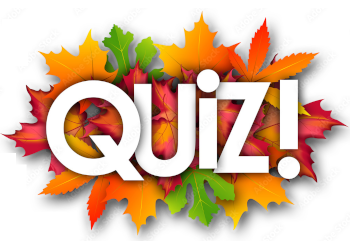
This quiz belongs to book/course code gs gs111 OOP Object Oriented Programming Java of gs organization. We have 178 quizzes available related to the book/course OOP Object Oriented Programming Java. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Types of Classes (Using Java). NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
class Base { void player1() { System.out.println("player1"); } } class Intermediate extends Base { void player2() { System.out.println("player2"); } } class SubClass extends Intermediate { void player3() { System.out.println("player3"); } public static void main(String args[]) { SubClass d=new SubClass(); d.player3(); } }
player3 player2 player1
player1 player2 player3
class Base { public void display() { System.out.println("Base class called"); } } class Derived extends Base { public void display() { System.out.println("Derived class called"); } public static void main(String[] args) { Base obj = new Derived(); obj.display(); } }
Base class called Derived class called
Derived class called Base class called
class Tier1 { public void Display() { System.out.println("Tier1"); } } class Tier2 extends Tier1 { public void Display() { System.out.println("Tier2"); } } class Tier3 extends Tier2 { public void Display() { super.super.Display(); System.out.println("Tier3"); } public static void main(String[] args) { Tier3 obj = new Tier3(); obj.Display(); } }
Tier3 Tier2 Tier1
class SampleClass1 { System.out.println("Class1"); } class SampleClass2 { System.out.println("Class2"); } class Base extends SampleClass2, SampleClass1 { void display() { System.out.println("Inherited"); } public static void main(String[] args) { Base obj = new Base(); obj.Display(); } }
Class2 Class1 Inherited
Inherited Class1 Class2
class Base { static void staticMethod() { System.out.println("Class X"); } } class Derived extends Base { static void staticMethod() { System.out.println("Class Y"); } public static void main(String[] args) { Base.staticMethod(); } }
Class X Class Y
class BaseClass { public static void main(String[] args) { String s = new String("HelloWorld"); Class strClass = s.getClass(); System.out.println("Base class of String: " + strClass.getSuperclass()); } }