gs gs111 Class Components (Using Java) - Data Members - Quiz No.1
gs gs111 OOP Object Oriented Programming Java Quiz
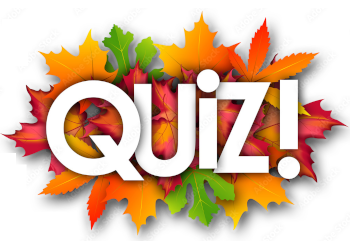
This quiz belongs to book/course code gs gs111 OOP Object Oriented Programming Java of gs organization. We have 178 quizzes available related to the book/course OOP Object Oriented Programming Java. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Class Components (Using Java). NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
class Books { abstract int price; Books(int p) { this.price = p; } public void getPrice() { System.out.println("Price: "+this.price); } } public class AbstractDataMembers { public static void main(String[] args) { Books b = new Books(20); b.getPrice(); } }
class Book { String title; String author; Book(String t) { this.title = t; } } public class InstanceMembers { public static void main(String[] args) { Book b = new Book("Java Programming"); System.out.println("Book Title: "+Book.title); } }
class Employee { int salary = 1000000; static float experience = 4.5f; } public class StaticDatamembers { public static void main(String[] args) { Employee e = new Employee(); System.out.println(e.experience); System.out.println(Employee.experience); } }
4.5 4.5
4.5 Compilation error
Compilation error 4.5
class Student { protected String name = "Java"; } class ProtectedVariables { public static void main(String[] args) { Student s = new Student(); System.out.println(s.name); } }
class Area { int side; public int squareArea() { int a = this.side * this.side; return a; } } public class InstanceVariables { public static void main(String[] args) { Area a = new Area(); System.out.println("Area: "+a.squareArea()); } }
class Distance { int time; static int speed = 10; static { int d = speed * time; } } public class StaticVariables { public static void main(String[] args) { Distance d = new Distance(); System.out.println("Speed: "+d.speed); System.out.println("Time: "+d.time); } }
Speed: 10 Time: 0
class Interest { int principal = 1000; int time = 2; static int rate = 3; static { Interest i = new Interest(); int interest = (i.principal * i.time * rate)/100; System.out.println("Simple Interest: "+interest); } } public class StaticMembers { public static void main(String[] args) { Interest i = new Interest(); } }