gs gs111 Types of Classes (Using Java) - Derived Class - Quiz No.1
gs gs111 OOP Object Oriented Programming Java Quiz
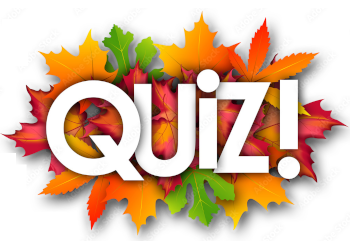
This quiz belongs to book/course code gs gs111 OOP Object Oriented Programming Java of gs organization. We have 178 quizzes available related to the book/course OOP Object Oriented Programming Java. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Types of Classes (Using Java). NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
class one { public void print1() { System.out.println("Hello"); } } class two extends one { public void print2() { System.out.println("World"); } } class Main { public static void main(String[] args) { two g = new two(); g.print2(); g.print1(); } }
World Hello
Hello World
World World Hello
class Super { public void pfunc() { System.out.println("Parent function"); } } class Sub extends Super { public void cfunc() { System.out.println("Child function"); } public static void main(String[] args) { Sub obj = new Sub(); obj.cfunc(); obj.pfunc(); } }
Child function Parent function
Parent function Child function
class Pulsar { String name; } class Bike extends Pulsar { String modelType; public void showDetail() { name = "Pulsar 2.0"; modelType = "Sports"; System.out.println(name); } public static void main(String[] args) { Bike car = new Bike(); car.showDetail(); } }
Pulsar 2.0 Sports
class Base { String name="base"; } class Derived extends Base { String name; public void details() { name = "Derived"; System.out.println(super.name+" and "+name); } public static void main(String[] args) { Derived obj = new Derived(); obj.details(); } }
class Person { public void show() { System.out.println("None"); } } class College extends Person { public void show1() { System.out.println("I am in a College"); } } class School extends Person { public void show2() { System.out.println("I am in a School"); } } class University extends Person { public void show3() { System.out.println("I am in a University"); } } class HierarchicalInheritance { public static void main(String args[]) { School teacher = new School(); College student = new College(); University doctor = new University(); student.show(); student.show1(); teacher.show2(); doctor.show3(); } }
None I am in a College I am in a School I am in a University
I am in a University I am in a School I am in a College None
class Base { void msg() { System.out.println("Hello"); } } class Intermediate { void msg() { System.out.println("Welcome"); } } class Derived extends Base,Intermediate { public static void main(String args[]) { C obj=new C(); obj.msg(); } }
Hello Welcome
Welcome Hello
class one { public void print_one() { System.out.print("one"); } } class two extends one { public void print_two() { System.out.print("two"); } public void print_one() { System.out.print("three"); } } class three extends two { public static void main(String[] args) { three obj = new three(); obj.print_one(); obj.print_two(); obj.print_one(); } }
class Line { public void print() { System.out.println("Inside display"); } } class Rectangle extends Line { public void area() { System.out.println("Inside area"); } } class Square extends Rectangle { public void volume() { System.out.println("Inside volume"); } } class Main { public static void main(String[] arguments) { Square obj = new Square(); obj.print(); } }
class Method1 { void fun() { System.out.println("Parent1"); } } class Method2 { void fun() { System.out.println("Parent2"); } } class Test extends Method1, Method2 { public static void main(String args[]) { Test t = new Test(); t.fun(); } }
Parent1 Parent2