gs gs109 Python OOPs - Python Inheritance - Quiz No.3
gs gs109 Python Quiz
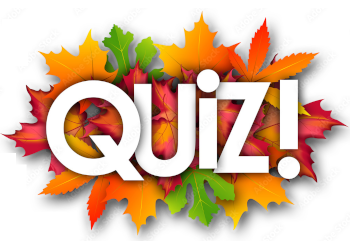
This quiz belongs to book/course code gs gs109 Python of gs organization. We have 134 quizzes available related to the book/course Python. This quiz has a total of 8 multiple choice questions (MCQs) to prepare and belongs to topic Python OOPs. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
Question 1: What will be the output of the following Python code?
>>> class A: pass >>> class B(A): pass >>> obj=B() >>> isinstance(obj,A)
True
False
Wrong syntax for isinstance() method
Invalid method for classes
Question 2: Which of the following statements is true?
The __new__() method automatically invokes the __init__ method
The __init__ method is defined in the object class
The __eq(other) method is defined in the object class
The __repr__() method is defined in the object class
Question 4: What will be the output of the following Python code?
class A: def __init__(self): self.__x = 1 class B(A): def display(self): print(self.__x) def main(): obj = B() obj.display() main()
1
0
Error, invalid syntax for object declaration
Error, private class member can’t be accessed in a subclass
Question 5: What will be the output of the following Python code?
class A: def __init__(self): self._x = 5 class B(A): def display(self): print(self._x) def main(): obj = B() obj.display() main()
Error, invalid syntax for object declaration
Nothing is printed
5
Error, private class member can’t be accessed in a subclass
Question 6: What will be the output of the following Python code?
class A: def __init__(self,x=3): self._x = x class B(A): def __init__(self): super().__init__(5) def display(self): print(self._x) def main(): obj = B() obj.display() main()
5
Error, class member x has two values
3
Error, protected class member can’t be accessed in a subclass
Question 7: What will be the output of the following Python code?
class A: def test1(self): print(" test of A called ") class B(A): def test(self): print(" test of B called ") class C(A): def test(self): print(" test of C called ") class D(B,C): def test2(self): print(" test of D called ") obj=D() obj.test()
test of B called test of C called
test of C called test of B called
test of B called
Error, both the classes from which D derives has same method test()
Question 8: What will be the output of the following Python code?
class A: def test(self): print("test of A called") class B(A): def test(self): print("test of B called") super().test() class C(A): def test(self): print("test of C called") super().test() class D(B,C): def test2(self): print("test of D called") obj=D() obj.test()
test of B called test of C called test of A called
test of C called test of B called
test of B called test of C called
Error, all the three classes from which D derives has same method test()