gs gs109 Python OOPs - Python Encapsulation - Quiz No.1
gs gs109 Python Quiz
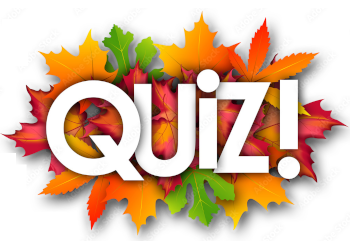
This quiz belongs to book/course code gs gs109 Python of gs organization. We have 134 quizzes available related to the book/course Python. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Python OOPs. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
Question 1: Which of these is not a fundamental features of OOP?
Encapsulation
Inheritance
Instantiation
Polymorphism
Question 2: Which of the following is the most suitable definition for encapsulation?
Ability of a class to derive members of another class as a part of its own definition
Means of bundling instance variables and methods in order to restrict access to certain class members
Focuses on variables and passing of variables to functions
Allows for implementation of elegant software that is well designed and easily modified
Question 3: What will be the output of the following Python code?
class Demo: def __init__(self): self.a = 1 self.__b = 1 def display(self): return self.__b obj = Demo() print(obj.a)
The program has an error because there isn’t any function to return self.a
The program has an error because b is private and display(self) is returning a private member
The program runs fine and 1 is printed
The program has an error as you can’t name a class member using __b
Question 4: What will be the output of the following Python code?
class Demo: def __init__(self): self.a = 1 self.__b = 1 def display(self): return self.__b obj = Demo() print(obj.__b)
The program has an error because there isn’t any function to return self.a
The program has an error because b is private and display(self) is returning a private member
The program has an error because b is private and hence can’t be printed
The program runs fine and 1 is printed
Question 5: Methods of a class that provide access to private members of the class are called as ______ and ______
getters/setters
__repr__/__str__
user-defined functions/in-built functions
__init__/__del__
Question 7: What will be the output of the following Python code?
class Demo: def __init__(self): self.a = 1 self.__b = 1 def get(self): return self.__b obj = Demo() print(obj.get())
The program has an error because there isn’t any function to return self.a
The program has an error because b is private and display(self) is returning a private member
The program has an error because b is private and hence can’t be printed
The program runs fine and 1 is printed
Question 8: What will be the output of the following Python code?
class Demo: def __init__(self): self.a = 1 self.__b = 1 def get(self): return self.__b obj = Demo() obj.a=45 print(obj.a)
The program runs properly and prints 45
The program has an error because the value of members of a class can’t be changed from outside the class
The program runs properly and prints 1
The program has an error because the value of members outside a class can only be changed as self.a=45