gs gs109 Python OOPs - Python Encapsulation - Quiz No.2
gs gs109 Python Quiz
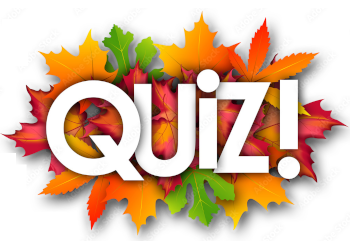
This quiz belongs to book/course code gs gs109 Python of gs organization. We have 134 quizzes available related to the book/course Python. This quiz has a total of 4 multiple choice questions (MCQs) to prepare and belongs to topic Python OOPs. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
Question 1: What will be the output of the following Python code?
class fruits: def __init__(self): self.price = 100 self.__bags = 5 def display(self): print(self.__bags) obj=fruits() obj.display()
The program has an error because display() is trying to print a private class member
The program runs fine but nothing is printed
The program runs fine and 5 is printed
The program has an error because display() can’t be accessed
Question 2: What will be the output of the following Python code?
class student: def __init__(self): self.marks = 97 self.__cgpa = 8.7 def display(self): print(self.marks) obj=student() print(obj._student__cgpa)
The program runs fine and 8.7 is printed
Error because private class members can’t be accessed
Error because the proper syntax for name mangling hasn’t been implemented
The program runs fine but nothing is printed
Question 3: Which of the following is false about protected class members?
They begin with one underscore
They can be accessed by subclasses
They can be accessed by name mangling method
They can be accessed within a class
Question 4: What will be the output of the following Python code?
class objects: def __init__(self): self.colour = None self._shape = "Circle" def display(self, s): self._shape = s obj=objects() print(obj._objects_shape)
The program runs fine because name mangling has been properly implemented
Error because the member shape is a protected member
Error because the proper syntax for name mangling hasn’t been implemented
Error because the member shape is a private member