gs gs116 Data Connectivity - LINQ - Quiz No.1
gs gs116 Microsoft SQL Server Quiz
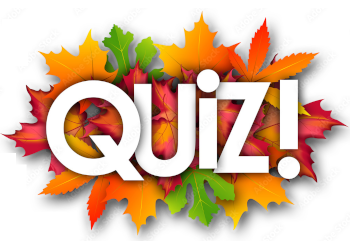
This quiz belongs to book/course code gs gs116 Microsoft SQL Server of gs organization. We have 100 quizzes available related to the book/course Microsoft SQL Server. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Data Connectivity. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
SELECT TOP 10 UPPER (c1.Name) FROM Customer c1 WHERE c1.Name LIKE 'A%' AND c1.ID NOT IN ( SELECT TOP 20 c2.ID FROM Customer c2 WHERE c2.Name LIKE 'A%' ORDER BY c2.Name ) ORDER BY c1.Name
var query = from c in db.Customers where c.Name.EndsWith ("A") orderby c.Name select c.Name.ToUpper(); var thirdPage = query.Skip(20).Take(10);
var query = from c in db.Customers where c.Name.StartsWith ("65") orderby c.Name select c.Name.ToUpper(); var thirdPage = query.Skip(20).Take(10);
var query = from c in db.Customers where c.Name.StartsWith ("A") orderby c.Name select c.Name.ToUpper(); var thirdPage = query.Skip(20).Take(10);
var query = from c in db.Customers where c.Name.StartsWith ("A") orderby c.Name select c.Name.ToLower(); var thirdPage = query.Skip(20).Take(10);
from c in db.Customers where c.Address.State == "WA" select new { c.Name, c.CustomerNumber, HighValuePurchases = c.Purchases.Where (p => p.Price > 1000) }
from c in db.Customers where c.Address.State = "WA" select new { c.Name, c.CustomerNumber, HighValuePurchases = c.Purchases.Where (p => p.Price > 1000) }
from c in db.Customers where c.Address.State == "WA" select new { c.Name, c.CustomerNumber, HighValuePurchases != c.Purchases.Where (p => p.Price > 1000) }
from p in db.Purchases where p.Customer.Address.State == "WA" || p.Customer == null where p.PurchaseItems.Sum (pi => pi.SaleAmount) = 1000 select p
from p in db.Purchases where p.Customer.Address.State == "WA" || p.Customer == null where p.PurchaseItems.Sum (pi => pi.SaleAmount) < 1000 select p
from p in db.Purchases where p.Customer.Address.State == "WA" || p.Customer == null where p.PurchaseItems.Sum (pi => pi.SaleAmount) > 1000 select p
public bool IsValidUser(string userName, string passWord) { DBNameDataContext myDB = new DBNameDataContext(); List<User> users = myDB.Users.Where(u => u.Username == userName && u.Password==passWord); if(users.Count>0) { return true; } return false; }
from p in db.Purchases where p.Customer.Address.State == "WA" || p.Customer == null where p.PurchaseItems.Sum (pi => pi.SaleAmount) > 1000 select p
SELECT p.* FROM Purchase p LEFT OUTER JOIN Customer c INNER JOIN Address a ON c.AddressID = a.ID ON p.CustomerID = c.ID WHERE (a.State = 'WA' || p.CustomerID IS NULL) AND p.ID in ( SELECT PurchaseID FROM PurchaseItem GROUP BY PurchaseID WHERE SUM (SaleAmount) > 1000 )
SELECT p.* FROM Purchase p LEFT OUTER JOIN Customer c INNER JOIN Address a ON c.AddressID = a.ID ON p.CustomerID = c.ID WHERE (a.State = 'WA' || p.CustomerID IS NULL) AND p.ID in ( SELECT PurchaseID FROM PurchaseItem GROUP BY PurchaseID HAVING SUM (SaleAmount) > 1000 )
SELECT p.* FROM Purchase p LEFT OUTER JOIN Customer c INNER JOIN Address a ON c.AddressID = a.ID ON p.CustomerID = c.ID WHERE (a.State = 'WA' || p.CustomerID IS NULL) AND p.ID in ( SELECT PurchaseID FROM PurchaseItem GROUP BY PurchaseID HAVING SUM (SaleAmount) < 1000 )
public bool IsValidUser(string userName, string passWord) { DBNameDataContext myDB = new DBNameDataContext(); return Enumerable.Count(userResults) > 0; }
public bool IsValidUser(string userName, string passWord) { DBNameDataContext myDB = new DBNameDataContext(); var userResults = from u in myDB.Users where u.Username == userName && u.Password == passWord select u; return Enumerable.Count(userResults) > 0; }
public bool IsValidUser(string userName, string passWord) { DBNameDataContext myDB = new DBNameDataContext(); var userResults = from u in myDB.Users where u.Username == userName && u.Password == passWord select u; return Enumerable.Count(userResults) == 0; }
foreach(User user in userResults) { //checking the result as like object if(user.Role == 'admin') { //do whatever you need } }
for(User user in userResults) { //checking the result as like object if(user.Role == 'admin') { //do whatever you need } }
While(User user in userResults) { //checking the result as like object if(user.Role == 'admin') { //do whatever you need } }
Online Quizzes of gs116 Microsoft SQL Server
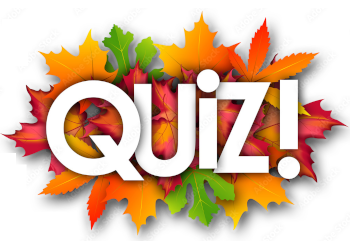
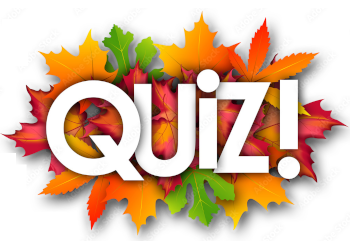
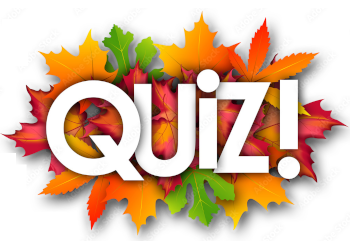
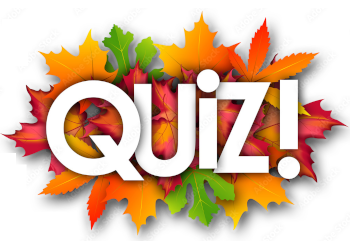