Which Of The Following Program Prints The Index Of Every #172
Which of the following program prints the index of every matching parenthesis?
This multiple choice question (MCQ) is related to the book/course
gs gs121 Data Structures and Algorithms.
It can also be found in
gs gs121 Application of Stacks - Decimal to Binary using Stacks - Quiz No.1.
Which of the following program prints the index of every matching parenthesis?
public void dispIndex(String exp) { Stack<Integer> stk = new Stack<Integer>(); for (int i = 0; i < len; i++) { char ch = exp.charAt(i); if (ch == '(') stk.push(i); else if (ch == ')') { try { int p = stk.pop() + 1; System.out.println("')' at index "+(i+1)+" matched with ')' at index "+p); } catch(Exception e) { System.out.println("')' at index "+(i+1)+" is unmatched"); } } } while (!stk.isEmpty() ) System.out.println("'(' at index "+(stk.pop() +1)+" is unmatched"); }
public void dispIndex(String exp) { Stack<Integer> stk = new Stack<Integer>(); for (int i = 0; i < len; i++) { char ch = exp.charAt(i); if (ch == '(') stk.push(i); else if (ch == ')') { try { int p = stk.pop() + 1; System.out.println("')' at index "+(i)+" matched with ')' at index "+p); } catch(Exception e) { System.out.println("')' at index "+(i)+" is unmatched"); } } } while (!stk.isEmpty() ) System.out.println("'(' at index "+(stk.pop() +1)+" is unmatched"); }
public void dispIndex(String exp) { Stack<Integer> stk = new Stack<Integer>(); for (int i = 0; i < len; i++) { char ch = exp.charAt(i); if (ch == ')') stk.push(i); else if (ch == '(') { try { int p = stk.pop() +1; System.out.println("')' at index "+(i+1)+" matched with ')' at index "+p); } catch(Exception e) { System.out.println("')' at index "+(i+1)+" is unmatched"); } } } while (!stk.isEmpty() ) System.out.println("'(' at index "+(stk.pop() +1)+" is unmatched"); }
public void dispIndex(String exp) { Stack<Integer> stk = new Stack<Integer>(); for (int i = 0; i < len; i++) { char ch = exp.charAt(i); if (ch == ')') stk.push(i); else if (ch == '(') { try { int p = stk.pop(); System.out.println("')' at index "+(i+1)+" matched with ')' at index "+p); } catch(Exception e) { System.out.println("')' at index "+(i+1)+" is unmatched"); } } } while (!stk.isEmpty() ) System.out.println("'(' at index "+(stk.pop() +1)+" is unmatched"); }
Similar question(s) are as followings:
Online Quizzes of gs121 Data Structures and Algorithms
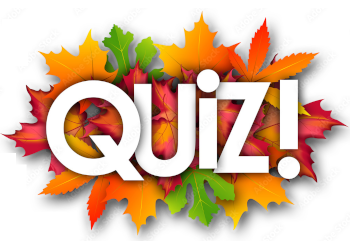
Binary Trees - Binary Search Tree - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes
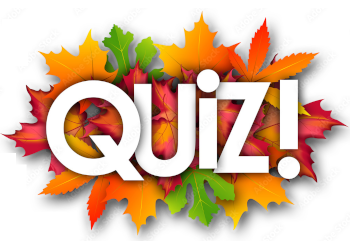
Binary Trees - Binary Search Tree - Quiz No.2
gs gs121 Data Structures and Algorithms
Online Quizzes
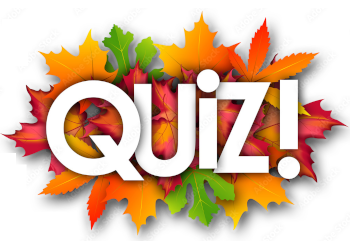
Binary Trees - Preorder Traversal - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes