gs gs121 Array Types - Sparse Array - Quiz No.1
gs gs121 Data Structures and Algorithms Quiz
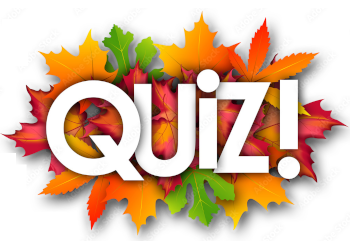
This quiz belongs to book/course code gs gs121 Data Structures and Algorithms of gs organization. We have 169 quizzes available related to the book/course Data Structures and Algorithms. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Array Types. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
public void store(int index, Object val) { List cur = this; List prev = null; List node = new List(index); node.val = val; while (cur != null && cur.index < index) { prev = cur; cur = cur.next; } if (cur == null) { prev.next = node; } else { if (cur.index == index) { System.out.println("DUPLICATE"); return; } prev.next = node; node.next = cur; } return; }
public void store(int index, Object val) { List cur = this; List prev = null; List node = new List(index); node.val = val; while (prev != null && prev.index < index) { prev = cur; cur = cur.next; } if (cur == null) { prev.next = node; } else { if (cur.index == index) { System.out.println("DUPLICATE"); return; } prev.next = node; node.next = cur; } return; }
public void store(int index, Object val) { List cur = this; List prev = null; List node = new List(index); node.val = val; while (cur != null && cur.index < index) { cur = cur.next; prev = cur; } if (cur == null) { prev.next = node; } else { if (cur.index == index) { System.out.println("DUPLICATE"); return; } prev.next = node; node.next = cur; } return; }
public void store(int index, Object val) { List cur = this; List prev = null; List node = new List(index); node.val = val; while (cur != null && prev.index < index) { cur = cur.next; prev = cur; } if (cur == null) { prev.next = node; } else { if (cur.index == index) { System.out.println("DUPLICATE"); return; } prev.next = cur; node.next = node; } return; }
public Object fetch(int index) { List cur = this.next; Object val = null; while (cur != null && cur.index != index) { cur = cur.next; } if (cur != null) { val = cur.val; } else { val = null; } return val; }
public Object fetch(int index) { List cur = this; Object val = null; while (cur != null && cur.index != index) { cur = cur.next; } if (cur != null) { val = cur.val; } else { val = null; } return val; }
public Object fetch(int index) { List cur = this; Object val = null; while (cur != null && cur.index != index) { cur = cur.index; } if (cur != null) { val = cur.val; } else { val = null; } return val; }
public Object fetch(int index) { List cur = this.next; Object val = null; while (cur != null && cur.index != index) { cur = cur.index; } if (cur != null) { val = cur.val; } else { val = null; } return val; }
public int count() { int count = 0; for (List cur = this.next; (cur != null); cur = cur.next) { count++; } return count; }
public int count() { int count = 0; for (List cur = this; (cur != null); cur = cur.next) { count++; } return count; }
public int count() { int count = 1; for (List cur = this.next; (cur != null); cur = cur.next) { count++; } return count; }
public int count() { int count = 1; for (List cur = this.next; (cur != null); cur = cur.next.next) { count++; } return count; }
public void store(int row_index, int col_index, Object val) { if (row_index < 0 || row_index > N) { System.out.println("row index out of bounds"); return; } if (col_index < 0 || col+index > N) { System.out.println("column index out of bounds"); return; } sparse_array[row_index].store(col_index, val); }
public void store(int row_index, int col_index, Object val) { if (row_index < 0 || row_index > N) { System.out.println("column index out of bounds"); return; } if (col_index < 0 || col+index > N) { System.out.println("row index out of bounds"); return; } sparse_array[row_index].store(col_index, val); }
public void store(int row_index, int col_index, Object val) { if (row_index < 0 && row_index > N) { System.out.println("row index out of bounds"); return; } if (col_index < 0 && col+index > N) { System.out.println("column index out of bounds"); return; } sparse_array[row_index].store(col_index, val); }
public void store(int row_index, int col_index, Object val) { if (row_index < 0 && row_index > N) { System.out.println("column index out of bounds"); return; } if (col_index < 0 && col+index > N) { System.out.println("row index out of bounds"); return; } sparse_array[row_index].store(col_index, val); }
public Object function(int row_index, int col_index) { if (row_index < 0 || col_index > N) { System.out.println("column index out of bounds"); return; } return (sparse_array[row_index].fetch(col_index)); }
Online Quizzes of gs121 Data Structures and Algorithms
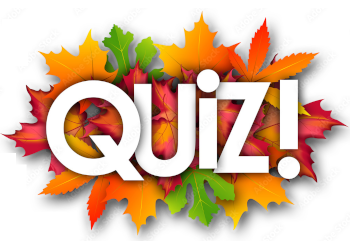
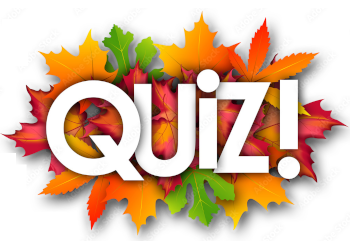
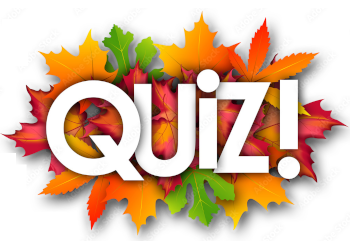