Given Below Is The Node Class To Perform Basic List Operations #119
Given below is the Node class to perform basic list operations and a Stack class with a no arg constructor. Select from the options the appropriate push() operation that can be included in the Stack class. Also ‘first’ is the top-of-the-stack.</p> <pre><code class="language-java"> class Node { protected Node next; protected Object ele; Node() { this(null,null); } Node(Object e,Node n) { ele=e; next=n; } public void setNext(Node n) { next=n; } public void setEle(Object e) { ele=e; } public Node getNext() { return next; } public Object getEle() { return ele; } } class Stack { Node first; int size=0; Stack() { first=null; } }</code></pre>
This multiple choice question (MCQ) is related to the book/course
gs gs121 Data Structures and Algorithms.
It can also be found in
gs gs121 Abstract Data Types - Stack using Linked List - Quiz No.1.
Given below is the Node class to perform basic list operations and a Stack class with a no arg constructor. Select from the options the appropriate push() operation that can be included in the Stack class. Also ‘first’ is the top-of-the-stack.
class Node { protected Node next; protected Object ele; Node() { this(null,null); } Node(Object e,Node n) { ele=e; next=n; } public void setNext(Node n) { next=n; } public void setEle(Object e) { ele=e; } public Node getNext() { return next; } public Object getEle() { return ele; } } class Stack { Node first; int size=0; Stack() { first=null; } }
public void push(Object item) { Node temp = new Node(item,first); first = temp; size++; }
public void push(Object item) { Node temp = new Node(item,first); first = temp.getNext(); size++; }
public void push(Object item) { Node temp = new Node(); first = temp.getNext(); first.setItem(item); size++; }
public void push(Object item) { Node temp = new Node(); first = temp.getNext.getNext(); first.setItem(item); size++; }
Similar question(s) are as followings:
Online Quizzes of gs121 Data Structures and Algorithms
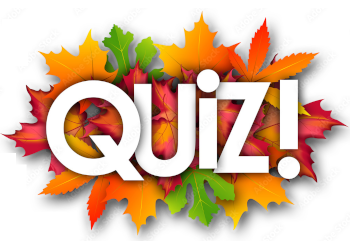
Binary Trees - Binary Search Tree - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes
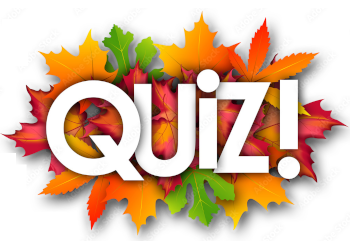
Binary Trees - Binary Search Tree - Quiz No.2
gs gs121 Data Structures and Algorithms
Online Quizzes
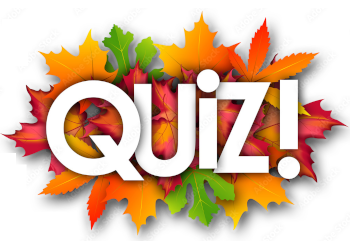
Binary Trees - Preorder Traversal - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes