gs gs108 Classes - Fundamentals of Class - Quiz No.1
gs gs108 CSharp Quiz
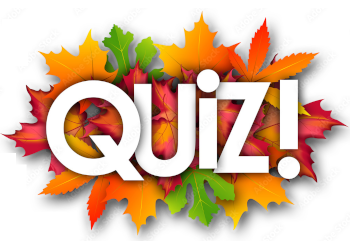
This quiz belongs to book/course code gs gs108 CSharp of gs organization. We have 110 quizzes available related to the book/course CSharp. This quiz has a total of 10 multiple choice questions (MCQs) to prepare and belongs to topic Classes. NVAEducation wants its users to help them learn in an easy way. For that purpose, you are free to prepare online MCQs and quizzes.
NVAEducation also facilitates users to contribute in online competitions with other students to make a challenging situation to learn in a creative way. You can create one to one, and group competition on an topic of a book/course code. Also on NVAEducation you can get certifications by passing the online quiz test.
Question 1: What will be the output of the following C# code?
class sample { public int i; public int[] arr = new int[10]; public void fun(int i, int val) { arr[i] = val; } } class Program { static void Main(string[] args) { sample s = new sample(); s.i = 10; sample.fun(1, 5); s.fun(1, 5); Console.ReadLine(); } }
sample.fun(1, 5) will not work correctly
s.i = 10 cannot work as i is ‘public’
sample.fun(1, 5) will set value as 5 in arr[1]
s.fun(1, 5) will work correctly
Question 2: Which of the following is used to define the member of a class externally?
:
::
#
none of the mentioned
Question 4: What is the most specified using class declaration?
type
scope
type & scope
none of the mentioned
Question 5: What will be the output of the following C# code?
class sample { public int i; public int j; public void fun(int i, int j) { this.i = i; this.j = j; } } class Program { static void Main(string[] args) { sample s = new sample(); s.i = 1; s.j = 2; s.fun(s.i, s.j); Console.WriteLine(s.i + " " + s.j); Console.ReadLine(); } }
Error while calling s.fun() due to inaccessible level
Error as ‘this’ reference would not be able to call ‘i’ and ‘j’
1 2
Runs successfully but prints nothing
Question 6: Which of the following statements about objects in “C#” is correct?
Everything you use in C# is an object, including Windows Forms and controls
Objects have methods and events that allow them to perform actions
All objects created from a class will occupy equal number of bytes in memory
All of the mentioned
Question 7: “A mechanism that binds together code and data in manipulates, and keeps both safe from outside interference and misuse. In short it isolates a particular code and data from all other codes and data. A well-defined interface controls access to that particular code and data.”
Abstraction
Polymorphism
Inheritance
Encapsulation
Question 8: What will be the output of the following C# code?
class z { public int X; public int Y; public const int c1 = 5; public const int c2 = c1 * 25; public void set(int a, int b) { X = a; Y = b; } } class Program { static void Main(string[] args) { z s = new z(); s.set(10, 20); Console.WriteLine(s.X + " " + s.Y); Console.WriteLine(z.c1 + " " + z.c2); Console.ReadLine(); } }
10 20 5 25
20 10 25 5
10 20 5 125
20 10 125 5
Question 9: Correct way of declaration of object of the following class is?
class name
name n = new name();
n = name();
name n = name();
n = new name();
Question 10: The data members of a class by default are?
protected, public
private, public
private
public