Consider The Following Dynamic Programming Implementation Of #2029
Consider the following dynamic programming implementation of the rod cutting problem:</p> <pre><code class="language-c"> #include<stdio.h> #include<limits.h> int rod_cut(int *prices, int len) { int max_val[len + 1]; int i,j,tmp_price,tmp_idx; max_val[0] = 0; for(i = 1; i <= len; i++) { int tmp_max = INT_MIN; // minimum value an integer can hold for(j = 1; j <= i; j++) { tmp_idx = i - j; //subtract 1 because index of prices starts from 0 tmp_price = _____________; if(tmp_price > tmp_max) tmp_max = tmp_price; } max_val[i] = tmp_max; } return max_val[len]; } int main() { int prices[]={2, 5, 6, 9, 9, 17, 17, 18, 20, 22},len_of_rod = 5; int ans = rod_cut(prices, len_of_rod); printf("%d",ans); return 0; }</code></pre> <p>Which line will complete the ABOVE code?
This multiple choice question (MCQ) is related to the book/course
gs gs122 Data Communication and Computer Network.
It can also be found in
gs gs122 Dynamic Programming - Rod Cutting - Quiz No.1.
Consider the following dynamic programming implementation of the rod cutting problem:
#include<stdio.h> #include<limits.h> int rod_cut(int *prices, int len) { int max_val[len + 1]; int i,j,tmp_price,tmp_idx; max_val[0] = 0; for(i = 1; i <= len; i++) { int tmp_max = INT_MIN; // minimum value an integer can hold for(j = 1; j <= i; j++) { tmp_idx = i - j; //subtract 1 because index of prices starts from 0 tmp_price = _____________; if(tmp_price > tmp_max) tmp_max = tmp_price; } max_val[i] = tmp_max; } return max_val[len]; } int main() { int prices[]={2, 5, 6, 9, 9, 17, 17, 18, 20, 22},len_of_rod = 5; int ans = rod_cut(prices, len_of_rod); printf("%d",ans); return 0; }
Which line will complete the ABOVE code?
prices[j-1] + max_val[tmp_idx]
prices[j] + max_val[tmp_idx]
prices[j-1] + max_val[tmp_idx - 1]
prices[j] + max_val[tmp_idx - 1]
Similar question(s) are as followings:
Online Quizzes of gs122 Data Communication and Computer Network
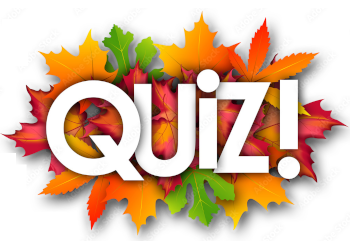
Sorting - Insertion Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
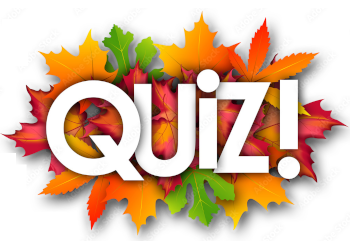
Sorting - Insertion Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes
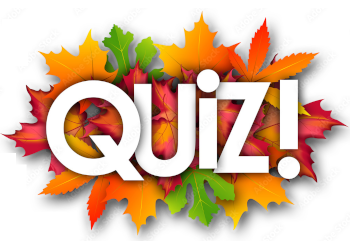
Sorting - Insertion Sort - Quiz No.3
gs gs122 Data Communication and Computer Network
Online Quizzes
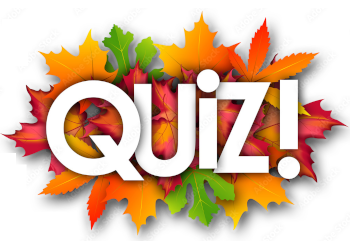
Sorting - LSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
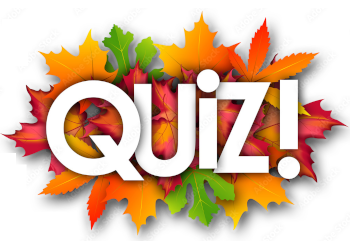
Sorting - MSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
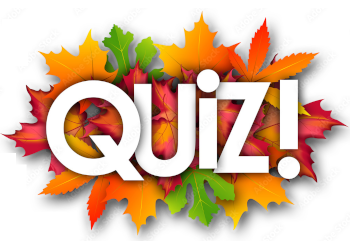
Sorting - MSD Radix Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes