Consider The Following Implementation Of Kadanes Algorithmp Pre #2008
Consider the following implementation of Kadane's algorithm:</p> <pre><code class="language-cpp"> 1. #include<stdio.h> 2. int max_num(int a, int b) 3. { 4. if(a > b) 5. return a; 6. return b; 7. } 8. int kadane_algo(int *arr, int len) 9. { 10. int ans = 0, sum = 0, idx; 11. for(idx =0; idx < len; idx++) 12. { 13. sum = max_num(0,sum + arr[idx]); 14. ans = max_num(sum,ans); 15. } 16. return ans; 17. } 18. int main() 19. { 20. int arr[] = {-2, -3, -3, -1, -2, -1, -5, -3},len = 8; 21. int ans = kadane_algo(arr,len); 22. printf("%d",ans); 23. return 0; 24. }</code></pre> <p>What changes should be made to the Kadane's algorithm so that it produces the right output even when all array elements are negative?</p> <pre><code class="language-cpp"> Change 1 = Line 10: int sum = arr[0], ans = arr[0] Change 2 = Line 13: sum = max_num(arr[idx],sum+arr[idx])</code></pre>
This multiple choice question (MCQ) is related to the book/course
gs gs122 Data Communication and Computer Network.
It can also be found in
gs gs122 Dynamic Programming - Kadane’s Algorithm - Quiz No.1.
Consider the following implementation of Kadane's algorithm:
1. #include<stdio.h> 2. int max_num(int a, int b) 3. { 4. if(a > b) 5. return a; 6. return b; 7. } 8. int kadane_algo(int *arr, int len) 9. { 10. int ans = 0, sum = 0, idx; 11. for(idx =0; idx < len; idx++) 12. { 13. sum = max_num(0,sum + arr[idx]); 14. ans = max_num(sum,ans); 15. } 16. return ans; 17. } 18. int main() 19. { 20. int arr[] = {-2, -3, -3, -1, -2, -1, -5, -3},len = 8; 21. int ans = kadane_algo(arr,len); 22. printf("%d",ans); 23. return 0; 24. }
What changes should be made to the Kadane's algorithm so that it produces the right output even when all array elements are negative?
Change 1 = Line 10: int sum = arr[0], ans = arr[0] Change 2 = Line 13: sum = max_num(arr[idx],sum+arr[idx])
Only Change 1 is sufficient
Only Change 2 is sufficient
Both Change 1 and Change 2 are necessary
No change is required
Similar question(s) are as followings:
Online Quizzes of gs122 Data Communication and Computer Network
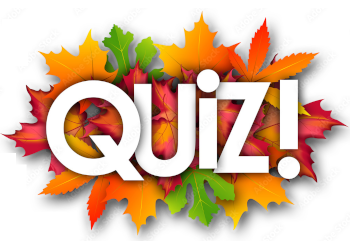
Sorting - Insertion Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
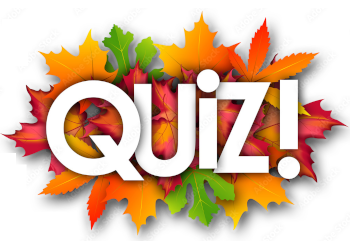
Sorting - Insertion Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes
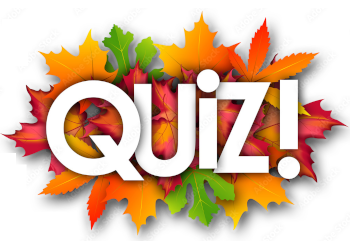
Sorting - Insertion Sort - Quiz No.3
gs gs122 Data Communication and Computer Network
Online Quizzes
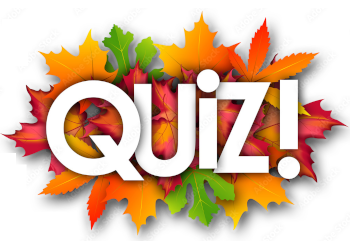
Sorting - LSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
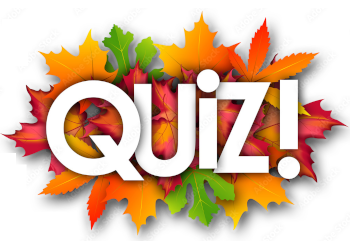
Sorting - MSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
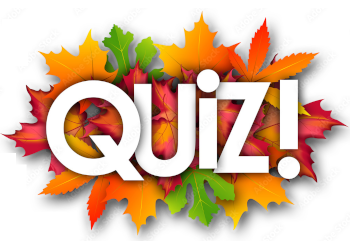
Sorting - MSD Radix Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes