Choose Correct C Code For Recursive Selection Sort From The #1769
Choose correct C++ code for recursive selection sort from the following.
This multiple choice question (MCQ) is related to the book/course
gs gs122 Data Communication and Computer Network.
It can also be found in
gs gs122 Recursion - Recursive Selection Sort - Quiz No.1.
Choose correct C++ code for recursive selection sort from the following.
#include <iostream> using namespace std; int minIndex(int a[], int i, int j) { if (i == 0) return i; int k = minIndex(a, i + 1, j); return (a[i] < a[k])? i : k; } void recursiveSelectionSort(int a[], int n, int index = 0) { if (index == n) return; int x = minIndex(a, index, n-1); if (x == index) { swap(a[x], a[index]); } recursiveSelectionSort(a, n, index + 1); } int main() { int arr[] = {5,3,2,4,1}; int n = sizeof(arr)/sizeof(arr[0]); recursiveSelectionSort(arr, n); return 0; }
#include <iostream> using namespace std; int minIndex(int a[], int i, int j) { if (i == j) return i; int k = minIndex(a, i + 1, j); return (a[i] < a[k])? i : k; } void recursiveSelectionSort(int a[], int n, int index = 0) { if (index == n) return; int x = minIndex(a, index, n-1); if (x != index) { swap(a[x], a[index]); } recursiveSelectionSort(a, n, index + 1); } int main() { int arr[] = {5,3,2,4,1}; int n = sizeof(arr)/sizeof(arr[0]); recursiveSelectionSort(arr, n); return 0; }
#include <iostream> using namespace std; int minIndex(int a[], int i, int j) { if (i == j) return i; int k = minIndex(a, i + 1, j); return (a[i] > a[k])? i : k; } void recursiveSelectionSort(int a[], int n, int index = 0) { if (index == n) return; int x = minIndex(a, index, n-1); if (x != index) { swap(a[x], a[index]); } recursiveSelectionSort(a, n, index + 1); } int main() { int arr[] = {5,3,2,4,1}; int n = sizeof(arr)/sizeof(arr[0]); recursiveSelectionSort(arr, n); return 0; }
#include <iostream> using namespace std; int minIndex(int a[], int i, int j) { if (i == j) return i; int k = minIndex(a, i + 1, j); return (a[i] > a[k])? i : k; } void recursiveSelectionSort(int a[], int n, int index = 0) { if (index == 0) return; int x = minIndex(a, index, n-1); if (x == index) { swap(a[x], a[index]); } recursiveSelectionSort(a, n, index + 1); } int main() { int arr[] = {5,3,2,4,1}; int n = sizeof(arr)/sizeof(arr[0]); recursiveSelectionSort(arr, n); return 0; }
Similar question(s) are as followings:
Online Quizzes of gs122 Data Communication and Computer Network
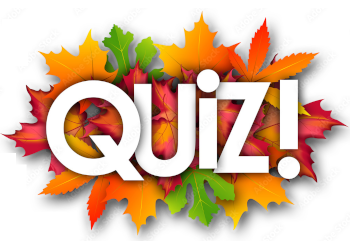
Sorting - Insertion Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
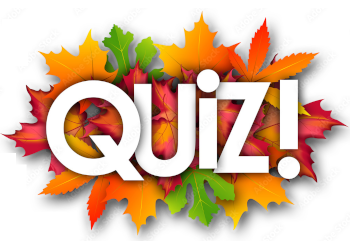
Sorting - Insertion Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes
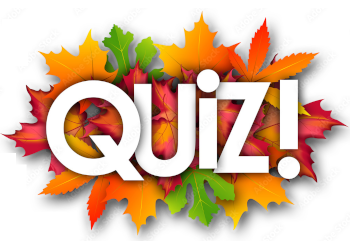
Sorting - Insertion Sort - Quiz No.3
gs gs122 Data Communication and Computer Network
Online Quizzes
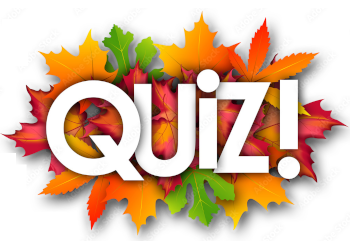
Sorting - LSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
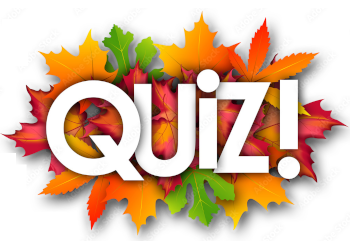
Sorting - MSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
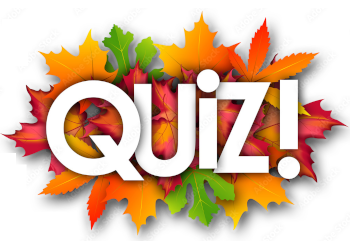
Sorting - MSD Radix Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes