Write A Function For The Fibonacci Search Method #500
Write a function for the Fibonacci search method.
This multiple choice question (MCQ) is related to the book/course
gs gs122 Data Communication and Computer Network.
It can also be found in
gs gs122 Searching - Fibonacci Search - Quiz No.1.
Write a function for the Fibonacci search method.
public static int fibSearch(final int key, final int[] a) { int low = 0; int high = a.length - 1; int fibCurrent = 1; int fibPrev = 1; int N = a.length; while (low <= high) { while(fibCurrent < N) { int tmp = fibCurrent + fibPrev; fibPrev = fibCurrent; fibCurrent = tmp; N = N - (fibCurrent - fibPrev); } final int mid = low + (high - low) - (fibCurrent + fibPrev); if (key < a[mid]) high = mid - 1; else if (key > a[mid]) low = mid + 1; else return mid; } return -1; }
public static int fibSearch(final int key, final int[] a) { int low = 0; int high = a.length - 1; int fibCurrent = 1; int fibPrev = 1; int N = a.length; while (low <= high) { int tmp = fibCurrent + fibPrev; fibPrev = fibCurrent; fibCurrent = tmp; N = N - (fibCurrent - fibPrev); final int mid = low + (high - low) - (fibCurrent + fibPrev); if (key < a[mid]) high = mid - 1; else if (key > a[mid]) low = mid + 1; else return mid; } return -1; }
public static int fibSearch(final int key, final int[] a) { int low = 0; int high = a.length - 1; int fibCurrent = 1; int fibPrev = 1; int N = a.length; while (low <= high) { while(fibCurrent < N) { int tmp = fibCurrent + fibPrev; fibPrev = fibCurrent; fibCurrent = tmp; N = N - (fibCurrent - fibPrev); } final int mid = low + (high - low) - (fibCurrent + fibPrev); if (key < a[mid]) low = mid + 1; else if (key > a[mid]) high = mid - 1; else return mid; } }
public static int fibSearch(final int key, final int[] a) { int low = 0; int high = a.length - 1; int fibCurrent = 1; int fibPrev = 1; int N = a.length; while (low <= high) { while(fibCurrent < N) { int tmp = fibCurrent + fibPrev; fibPrev = fibCurrent; fibCurrent = tmp; N = N - (fibCurrent - fibPrev); } final int mid = low + (high - low) - (fibCurrent + fibPrev); if (key < a[mid]) low = mid - 1; else if (key > a[mid]) high = mid - 1; else return mid; } }
Similar question(s) are as followings:
Online Quizzes of gs122 Data Communication and Computer Network
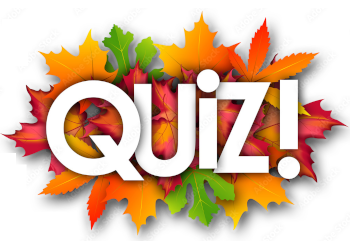
Sorting - Insertion Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
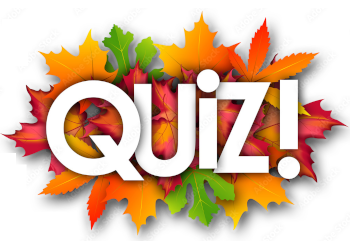
Sorting - Insertion Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes
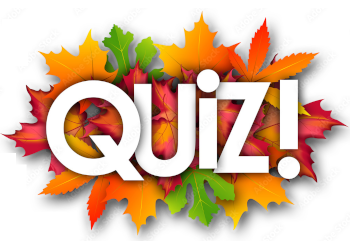
Sorting - Insertion Sort - Quiz No.3
gs gs122 Data Communication and Computer Network
Online Quizzes
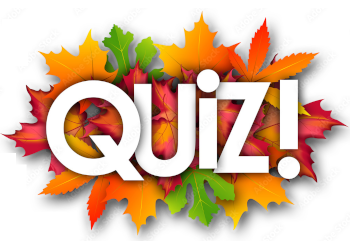
Sorting - LSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
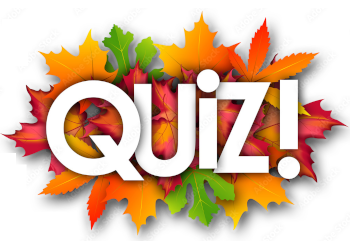
Sorting - MSD Radix Sort - Quiz No.1
gs gs122 Data Communication and Computer Network
Online Quizzes
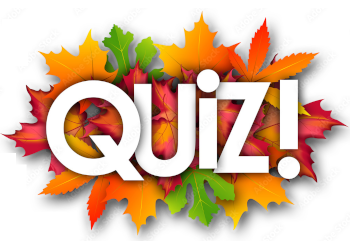
Sorting - MSD Radix Sort - Quiz No.2
gs gs122 Data Communication and Computer Network
Online Quizzes