Select The Appropriate Code Which Tests For A Palindrome #260
Select the appropriate code which tests for a palindrome.
This multiple choice question (MCQ) is related to the book/course
gs gs121 Data Structures and Algorithms.
It can also be found in
gs gs121 Application of Stacks - Towers of Hanoi - Quiz No.1.
Select the appropriate code which tests for a palindrome.
public static void main(String[] args) { System.out.print("Enter any string:"); Scanner in=new Scanner(System.in); String input = in.nextLine(); Stack<Character> stk = new Stack<Character>(); for (int i = 0; i < input.length(); i++) { stk.push(input.charAt(i)); } String reverse = ""; while (!stk.isEmpty()) { reverse = reverse + stk.pop(); } if (input.equals(reverse)) System.out.println("palindrome"); else System.out.println("not a palindrome"); }
public static void main(String[] args) { System.out.print("Enter any string:"); Scanner in=new Scanner(System.in); String input = in.nextLine(); Stack<Character> stk = new Stack<Character>(); for (int i = 0; i < input.length(); i++) { stk.push(input.charAt(i)); } String reverse = ""; while (!stk.isEmpty()) { reverse = reverse + stk.peek(); } if (input.equals(reverse)) System.out.println("palindrome"); else System.out.println("not a palindrome"); }
public static void main(String[] args) { System.out.print("Enter any string:"); Scanner in=new Scanner(System.in); String input = in.nextLine(); Stack<Character> stk = new Stack<Character>(); for (int i = 0; i < input.length(); i++) { stk.push(input.charAt(i)); } String reverse = ""; while (!stk.isEmpty()) { reverse = reverse + stk.pop(); stk.pop(); } if (input.equals(reverse)) System.out.println("palindrome"); else System.out.println("not a palindrome"); }
public static void main(String[] args) { System.out.print("Enter any string:"); Scanner in=new Scanner(System.in); String input = in.nextLine(); Stack<Character> stk = new Stack<Character>(); for (int i = 0; i < input.length(); i++) { stk.push(input.charAt(i)); } String reverse = ""; while (!stk.isEmpty()) { reverse = reverse + stk.pop(); stk.pop(); } if (!input.equals(reverse)) System.out.println("palindrome"); else System.out.println("not a palindrome"); }
Similar question(s) are as followings:
Online Quizzes of gs121 Data Structures and Algorithms
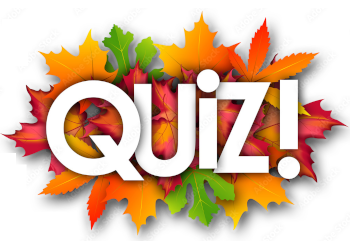
Binary Trees - Binary Search Tree - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes
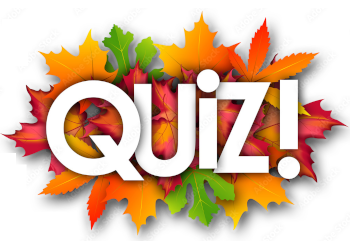
Binary Trees - Binary Search Tree - Quiz No.2
gs gs121 Data Structures and Algorithms
Online Quizzes
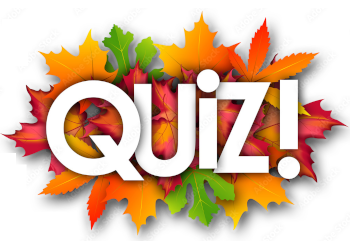
Binary Trees - Preorder Traversal - Quiz No.1
gs gs121 Data Structures and Algorithms
Online Quizzes